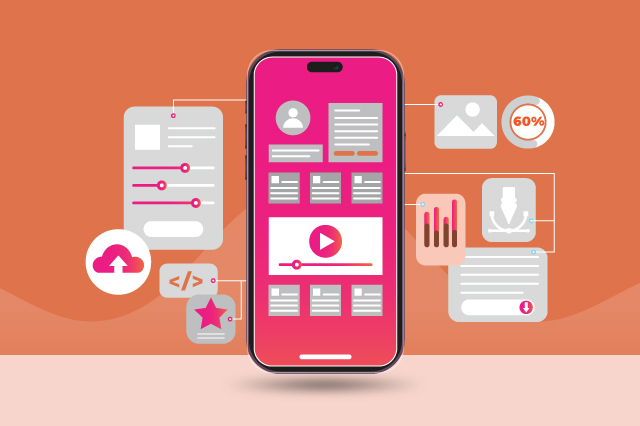
As we know, FlutterFlow is a visual development platform designed for building Flutter apps, does not natively offer features or plugins for implementing background services on both Android and iOS. And it focuses on simplifying the app development process.
However, you can achieve background service in your FlutterFlow app by using Flutter plugins within your FlutterFlow project. We have many Flutter plugins like background_fetch, flutter_background_service, to handle background tasks. You can easily achieve this service for android. But for iOS it is a bit complicated.
In my recent project which involved periodic background tasks, specifically fetching location data and sending it to an API, I have done much research on how to implement this in flutterflow but I didn’t get any solution. After many research i got one solution which i’m going to share here.
This approach proved successful, especially when faced with the specific requirement of fetching location data in the background. Notably, other packages did not offer the desired level of compatibility or support within the FlutterFlow context. It’s important to note that the implementation of background functionality, particularly on iOS, may pose challenges and may not be as straightforward.
To fetch location and run it in background i have used two packages:
1. Geolocator: This package facilitates the retrieval of location data.
2. Flutter_background_service: This package enables the execution of the application in the background.
In conclusion, the combination of the geolocator and flutter_background_service packages offer a viable solution for implementing background functionality within a FlutterFlow project. It is recommended to closely adhere to the outlined steps and continually follow the below steps:
Step 1: Download Project Code
Download your FlutterFlow project code and open it in a text editor, such as Visual Studio Code.
Step 2: Implement Background Service
Refer to the Flutter documentation for background tasks and specifically, utilize the flutter_background_service package. Detailed information can be found on the official package page: https://pub.dev/packages/flutter_background_service.
Step 3: Configure Dependencies
To enable background service functionality on iOS, add the following dependencies to your pubspec.yaml file. Ensure you are using the latest versions:
dependencies:
flutter_background_service: ^5.0.5
geolocator: ^10.1.0
http: ^0.13.5
device_info_plus: ^9.1.1
flutter_local_notifications: ^16.3.0 # Optional for notification functionality
permission_handler: 10.0.0
Run flutter pub get to fetch the latest dependencies.
Step 4: Fetch Location Code
Write code to fetch the device’s location. Refer to the geolocator package documentation for guidance: https://pub.dev/packages/geolocator.
Step 5: Background Service Code
Implement the background service code using the flutter_background_service package. Follow the documentation provided here: https://pub.dev/packages/flutter_background_service.
Step 6: Combine Location and Background Service Code
Ensure that you consolidate the code for fetching location and the background service within a single file.
Step 7: Integrate Background Service
Call your background service method from within your main widget file to initiate the background service. This ensures that the background tasks are triggered appropriately.
Now we have to do some configuration for ios
1. Make sure all required dependencies are added to your pubspec.yaml file. Run flutter pub get to fetch the latest versions.
2. Add Permissions in Info.plist
Inside the Info.plist file, add the necessary permissions for location access and background operation:
3. Now open AppDelegate.swift file in your vs code and add these lines
import flutter_background_service_ios // add this
SwiftFlutterBackgroundServicePlugin.taskIdentifier =”com.Example.background.refresh”
Make sure to add the same task identifire in your info.plist file under “BGTaskSchedulerPermittedIdentifiers” key.
4. Whatever permission you have given in your info.plist same permission should be there in your Podfile also.
Make sure flutter SDK is installed in your macOS.
Now open your ios folder inside xcode.
Turning on the Background Modes capability
1. Go to Xcode and open your project.
2. In your app target, navigate to Capabilities tab.
3. Turn on Background Modes.
Please turn on location update, background fetch, remote notification and background processing to enable background service for iOS. If you will not turn on these modes, then your application will crash.
That’s it… Now you can build your app to TestFlight from Xcode.
About Author
Gulshan Kumar Patel is a Software Engineer at Chimera Technologies. He specializes in full-stack development, proficient in using technologies like Java, Spring Boot, Hibernate, and React Js for front-end development. In addition to his expertise in web development, Gulshan is also interested in mobile application development, employing modern frameworks such as Flutter and no-code/low-code tools like FlutterFlow. His diverse skill set enables him to contribute to projects across multiple platforms, driving innovation and efficiency.